Danh sách thành viên | Cá nhân | Nhà đất, bất động sản

Diễn đàn
ASP.NET & Sharepoint MOSS, WSS 2007
Thư viện FTPClient chuẩn Thành viên | Nội dung | aspnet
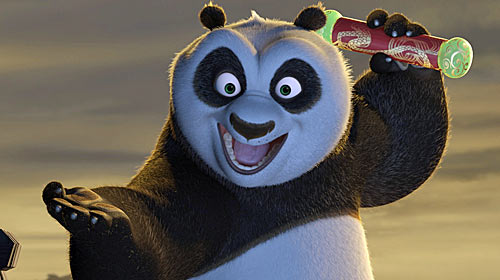 Lập trình không biên giới 604 bài
| public class FTPClient { private string remoteHost; private string remoteUser; private string remotePass; private string remotePath;
private int remotePort = 21; private int bytes; private Socket clientSocket;
private int retValue; private Boolean logined; private string reply; private string mes = "";
private static int BLOCK_SIZE = 512;
Byte [] buffer = new Byte [BLOCK_SIZE] ; Encoding ASCII = Encoding.ASCII;
public FTPClient(string host, string user, string password) { remoteHost = host; remoteUser = user; remotePass = password; Login(); }
public void Login() { clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); IPEndPoint ep = new IPEndPoint(Dns.Resolve(remoteHost).AddressList [0] , remotePort);
try { clientSocket.Connect(ep); } catch (Exception) { throw new IOException("Couldn't connect to remote server"); }
ReadReply(); if (retValue != 220) { Close(); throw new IOException(reply.Substring(4)); }
SendCommand("USER " + remoteUser); if (!(retValue == 331 || retValue == 230)) { CleanUp(); throw new IOException(reply.Substring(4)); }
if (retValue != 230) { SendCommand("PASS " + remotePass); if (!(retValue == 230 || retValue == 202)) { CleanUp(); throw new IOException(reply.Substring(4)); } }
logined = true; }
private void ChangeDir(string dirName) { if (dirName.Equals(".")) { return; }
SendCommand("CWD " + dirName);
if (retValue != 250) { throw new IOException(reply.Substring(4)); }
this.remotePath = dirName; }
private void ReadReply() { mes = ""; reply = ReadLine(); retValue = Int32.Parse(reply.Substring(0, 3)); }
private string ReadLine() { while (true) { bytes = clientSocket.Receive(buffer, buffer.Length, 0); mes += ASCII.GetString(buffer, 0, bytes); if (bytes < buffer.Length) { break; } }
string [] messages = mes.Split('\n');
if (mes.Length > 2) { mes = messages [messages.Length - 2] ; } else { mes = messages [0] ; }
if (!mes.Substring(3, 1).Equals(" ")) { return ReadLine(); }
return mes; }
private void SendCommand(string command) { Byte [] cmdBytes = Encoding.ASCII.GetBytes((command + "\r\n").ToCharArray()); clientSocket.Send(cmdBytes, cmdBytes.Length, 0); ReadReply(); }
public void Close() { if (clientSocket != null) { SendCommand("QUIT"); }
CleanUp(); }
public void CleanUp() { if (clientSocket != null) { clientSocket.Close(); clientSocket = null; } logined = false; }
public void UploadFile(string fileName, Stream filePosted) { Socket cSocket = CreateDataSocket(); //long offset = 0;
//if (resume) //{ // try // { // SetBinaryMode(true); // offset = getFileSize(fileName); // } // catch (Exception) // { // offset = 0; // } //}
//if (offset > 0) //{ // SendCommand("REST " + offset); // if (retValue != 350) // { // offset = 0; // } //}
SendCommand("STOR " + fileName);
if (!(retValue == 125 || retValue == 150)) { throw new IOException(reply.Substring(4)); }
while ((bytes = filePosted.Read(buffer, 0, buffer.Length)) > 0) { cSocket.Send(buffer, bytes, 0); }
filePosted.Close(); if (cSocket.Connected) { cSocket.Close(); }
ReadReply(); if (!(retValue == 226 || retValue == 250)) { throw new IOException(reply.Substring(4)); } }
private Socket CreateDataSocket() { SendCommand("PASV");
if (retValue != 227) { throw new IOException(reply.Substring(4)); }
int index1 = reply.IndexOf('('); int index2 = reply.IndexOf(')'); string ipData = reply.Substring(index1 + 1, index2 - index1 - 1); int [] parts = new int [6] ;
int len = ipData.Length; int partCount = 0; string buf = "";
for (int i = 0; i < len && partCount <= 6; i++) {
char ch = Char.Parse(ipData.Substring(i, 1)); if (Char.IsDigit(ch)) buf += ch; else if (ch != ',') { throw new IOException("Malformed PASV reply: " + reply); }
if (ch == ',' || i + 1 == len) {
try { parts [partCount++] = Int32.Parse(buf); buf = ""; } catch (Exception) { throw new IOException("Malformed PASV reply: " + reply); } } }
string ipAddress = parts [0] + "." + parts [1] + "." + parts [2] + "." + parts [3] ;
int port = (parts [4] << 8) + parts [5] ;
Socket s = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); IPEndPoint ep = new IPEndPoint(Dns.Resolve(ipAddress).AddressList [0] , port);
try { s.Connect(ep); } catch (Exception) { throw new IOException("Can't connect to remote server"); }
return s; }
private void SetBinaryMode(bool mode) { if (mode) { SendCommand("TYPE I"); } else { SendCommand("TYPE A"); }
if (retValue != 200) { throw new IOException(reply.Substring(4)); } } }
Chia sẻ với bà con, cái này được viết trên java, có 1 chú rất chăm chỉ chuyển sang dotnet, chạy ổn định gần như không có lỗi   
|  |
Chủ đề gần đây :
Cùng loại :
Tên file
|
Người đăng
|
Ngày
|
Lượt
|
vspforum.zip
Ma nguon vspforum ngay xua
|
aspnet |
4/18/2023 6:38:37 AM |
8 |
pdfjs.rar
pdfjs 2017 : hiển thị tốt trên iphone 11, 12, 13 không lỗi, bản 2012 sẽ lỗi trên iphone
|
aspnet |
6/21/2022 11:52:48 AM |
2 |
pdfjs2.rar
Xem file pdf bằng viewer.hml cua pdfjs (thư viện chuẩn mozilla) 2012. https://mozilla.github.io/pdf.js/getting_started/#download có thể download bản prebuild tại đây
|
aspnet |
6/21/2022 11:52:04 AM |
2 |
runner.zip
using three.js, orbitcontrol to view an object move random on map. Di chuyển 1 đồ vật ngẫu nhiên trên bản đồ, sử dụng với demo nhân viên di chuyển trong văn phòng. Toàn js download về là chạy
|
aspnet |
12/5/2019 5:55:14 PM |
0 |
gmap.zip
google map + marker
|
aspnet |
7/17/2019 2:25:05 PM |
1 |
vinsmarthomeservice.zip
java post json to api, use AsyncTask, event listener
|
aspnet |
7/9/2019 5:00:10 PM |
1 |
fblogin.zip
Login facebook bang javascript SDK
|
aspnet |
7/9/2019 9:16:37 AM |
0 |
autocomplete-location.zip
autocomplete location geo from google place, html + js
|
aspnet |
7/4/2019 4:37:55 PM |
2 |
WebAPI.zip
api for android access db (v1.0.0)
|
aspnet |
7/4/2019 9:14:17 AM |
8 |
KydientuPdf.zip
Ky dien tu file PDF su dung itextsharp
|
aspnet |
4/9/2019 3:30:37 PM |
9 |
GooglePlusLogin.zip
Login Google Plus account, C#, web asp.net ver2.0. Simple connect google APIs. Send key, get token, get full account info
|
aspnet |
6/1/2018 10:41:12 AM |
11 |
WebApplication1.rar
Sample su dung thuat toan ma hoa tripDES, co khoa bi mat (privateKey)
|
aspnet |
3/30/2018 10:06:35 PM |
8 |
NETMdbToolsTestApp.rar
dotNet MdbTools for Access 2003/2007/2016 without Microsoft Jet Engine, source C#, https://www.codeproject.com/Articles/283626/MsAccess-MdbTools-with-MFC-and-NET
|
aspnet |
3/26/2018 11:43:16 PM |
1 |
Cryptography_MD5_TriDES_src.zip
Thuật toán mã hóa 2 chiều TriDES, gồm Encrypt và Decrypt, aspnet 2.0
|
aspnet |
3/22/2018 11:20:44 AM |
3 |
mvc.rar
sample project MVC on C#
|
aspnet |
3/20/2018 9:25:36 AM |
9 |
EduPortal.rar
Edu portal frame work for VB.NET
|
aspnet |
3/14/2018 12:00:41 AM |
13 |
AutoEntity.rar
Gencode vb.net visual studio 2015. dotnet v2.0
|
aspnet |
3/13/2018 11:59:16 PM |
2 |
GenCode.rar
Gencode XML, XSLT, Info, DAL .. engine enterprise for quick app database
|
aspnet |
2/5/2018 9:37:28 AM |
9 |
DataXml.rar
Read DB from SQL to XML file, Convert string TCVN to Unicode
|
aspnet |
1/29/2018 2:15:45 PM |
4 |
DesktopModules.rar
Module quản lý tin tức, CMS, quản lý nhóm tin trên dotnetnuke 6.x
|
aspnet |
3/7/2013 4:47:49 PM |
1715 |
|